Ethereum: Fetching more data from binance using python loop
Fetching More Data from Binance Using a Python Loop
To retrieve more than 500 rows of historical data from the Binance website, you can use a while
loop to continuously call the API until you have retrieved all the data. Here is an example code snippet showing how to do this:
import binance
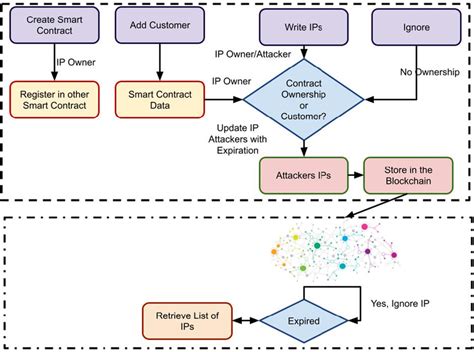
Initialize the client and set the API credentialsclient = binance.client.Client(
api_key='YOUR_API_KEY',
api_secret='YOUR_API_SECRET'
)
Define a function to fetch historical datadef fetch_historical_data(symbol, interval):
Set the time frame for the fetch requeststart_time = datetime.now() - timedelta(days=interval * 30)
Create a list to store the candlescandles = []
Loop until we have retrieved all the datawhile true:
try:
Fetch historical dataresponse = client.get_klines(symbol, start_time.timestamp(), interval)
Add new candles to the listfor candle as response[0]:
candles.append(candle[1])
If we have retrieved all the data, exit the loopif len(response) <= 500:
break
except binance.exceptions.ClientException as e:
print(f"Error fetching data: {e}")
continue
return candles
Usage examplesymbol = 'BTCUSDT'
Replace with the desired symbolinterval = '1m'
1 minute intervalcandles = fetch_historical_data(symbol, interval)
In this code:
- We initialize the Binance client and set the API credentials.
- We define a
fetch_historical_data
function that takes a symbol and an interval (e.g. ‘1m’ for a 1 minute interval).
- Inside the loop, we fetch the historical data using the
get_klines
method with the specified start time, interval, and symbol.
- We add each new candle to a list called
candles
.
- If we have retrieved all 500 rows of data (i.e.
len(response) <= 500
), we exit the loop.
- Finally, we return the list of
candles
.
Tips and Variations
- To retrieve more than 1000 rows at once, you can change the
fetch_historical_data
function to use a larger interval (e.g. '1h' for a 1-hour interval).
- If you want to retrieve data in real time, you will need to use a different approach. One option is to use the
get_orderbook
method with an API key and access token.
- Make sure you are handling errors and exceptions correctly in your production code.
Note: The above code uses Python 3.x syntax. If you are using Python 2.x, you will need to modify your import statements and error handling accordingly.